初始化脚手架
🦋 Vue CLI官网:https://cli.vuejs.org/zh/
第一步(仅第一次执行):全局安装@vue/cli
。使用命令npm install -g @vue/cli
。
安装成功后可使用vue --version
查看当前版本。
升级全局的Vue CLI,使用npm update -g @vue/cli
可升级最新版CLI。
第二步:切换到你要创建项目的目录
,使用vue create xxx
(xxx为项目名字)创建项目 or 使用图形化界面命令vue ui
创建和管理项目
第三步: 启动项目npm run serve
注:
1.配置npm淘宝镜像:npm config set registry https://registry.npmmirror.com/
(npm config get registry
查看npm镜像)
2.CLI服务:默认 preset 的项目的 package.jso
n:
{
“scripts”: {
“serve”: “vue-cli-service serve”,
“build”: “vue-cli-service build”
}
}
用法:vue-cli-service serve [options] [entry]
选项:
--open
在服务器启动时打开浏览器
--copy
在服务器启动时将 URL 复制到剪切版
--mode
指定环境模式 (默认值:development)
--host
指定 host (默认值:0.0.0.0)
--port
指定 port (默认值:8080)
--https
使用 https (默认值:false)
例: "serve": "vue-cli-service serve --open"
关于不同版本的Vue
vue.js
与vue.runtime.xxx.js
的区别:
vue.js
是完整版的Vue,包含:核心功能
+ 模板解析器
。
vue.runtime.xxx.js
是运行版的Vue,只包含:核心功能
;没有模板解析器。
- 因为vue.runtime.xxx.js没有模板解析器,所以不能使用
template
这个配置项,需要使用render函数
接收到的createElement函数
去指定具体内容。
Vue.config.js配置文件
- Vue脚手架隐藏了所有webpack相关的配置,若想查看具体的
webpack
配置,执行:vue inspect > output.js
- 使用vue.config.js可以对脚手架进行个性化定制,详情见:https://cli.vuejs.org/zh
项目结构
├── node_modules
├── public
│ ├── favicon.ico: 页签图标
│ └── index.html: 主页面
├── src
│ ├── assets: 存放静态资源
│ │ └── logo.png
│ │── component: 存放组件
│ │ └── School.vue
│ │ └── Student.vue
│ │── App.vue: 汇总所有组件
│ │── main.js: 入口文件
├── .gitignore: git 版本管制忽略的配置
├── babel.config.js: babel 的配置文件
├── package.json: 应用包配置文件
├── README.md: 应用描述文件
├── package-lock.json:包版本控制文
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <template> <div class="demo"> <h2>学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> <button @click="showName">点我提示学校名</button> </div> </template>
<script> export default { name:'School', data(){ return { name:'ay', address:'北京昌平' } }, methods: { showName(){ alert(this.name) } }, } </script>
<style> .demo{ background-color: orange; } </style>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <template> <div> <h2>学生姓名:{{name}}</h2> <h2>学生年龄:{{age}}</h2> </div> </template>
<script> export default { name:'Student', data(){ return { name:'张三', age:18 } } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22
| <template> <div> <img src="./assets/logo.png" alt="logo"> <School></School> <Student></Student> </div> </template>
<script> import School from './components/School' import Student from './components/Student'
export default { name:'App', components:{ School, Student } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
|
import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({ el: '#app', render: h => h(App), })
|
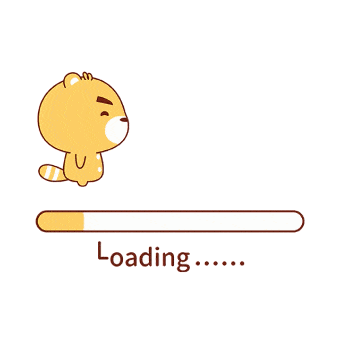
ref属性
🚀ref
被用来给元素
或子组件
注册引用信息(给节点打标识!)。引用信息将会注册在父组件
的 $refs
对象上。如果在普通的 DOM 元素上使用,引用指向的就是 DOM 元素
;如果用在子组件
上,引用就指向组件实例
。
当 v-for
用于元素或组件的时候,引用信息将是包含 DOM 节点
或组件实例
的数组
。
关于 ref 注册时间的重要说明:因为ref
本身是作为渲染结果被创建的,在初始渲染
的时候你不能访问它们。它们还不存在
!$refs
也不是响应式
的,因此你不应该试图用它在模板中做数据绑定
。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24
| <template> <div class="school"> <h2>学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> </div> </template>
<script> export default { name:'School', data() { return { name:'ay', address:'北京·昌平' } }, } </script>
<style> .school{ background-color: gray; } </style>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| <template> <div> <h1 v-text="msg" ref="title"></h1> <button ref="btn" @click="showDOM">点我输出上方的DOM元素</button> <School ref="sch"/> </div> </template>
<script> import School from './components/School'
export default { name:'App', components:{School}, data() { return { msg:'欢迎学习Vue!' } }, methods: { showDOM(){ console.log(this.$refs.title) console.log(this.$refs.btn) console.log(this.$refs.sch) } }, } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({ el:'#app', render: h => h(App) })
|
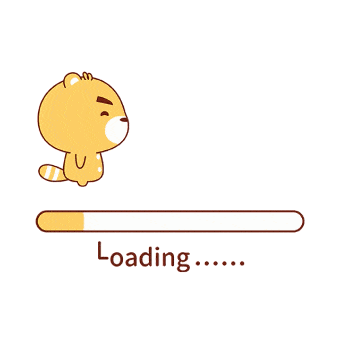
props配置项
🐋props配置项
(ps:个人理解:类似微信转账,需要对方接收💴才可以到账)
- 功能:让组件接收外部传过来的数据
- 传递数据:
<Demo name="xxx"/>
- 接收数据:
- 第一种方式(只接收):
props:['name']
- 第二种方式(限制类型):
props:{name:String}
- 第三种方式(限制类型、限制必要性、指定默认值):
1 2 3 4 5 6 7
| props:{ name:{ type:String, required:true, default:'老王' } }
|
备注:props是只读
的,Vue底层会监测你对props的修改
,如果进行了修改,就会发出警告,若业务需求确实需要修改,那么请复制props的内容到data中一份,然后去修改data中的数据(ps:个人建议不要对props进行修改,有点违反原则)。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| <template> <div> <Student name="李四" sex="女" :age="18"/> </div> </template>
<script> import Student from './components/Student'
export default { name:'App', components:{Student} } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| <template> <div> <h1>{{msg}}</h1> <h2>学生姓名:{{name}}</h2> <h2>学生性别:{{sex}}</h2> <h2>学生年龄:{{myAge+1}}</h2> <button @click="updateAge">尝试修改收到的年龄</button> </div> </template>
<script> export default { name:'Student', data() { console.log(this) return { msg:'我是一个育才的学生', myAge:this.age } }, methods: { updateAge(){ this.myAge++ } },
props:{ name:{ type:String, required:true, }, age:{ type:Number, default:99 }, sex:{ type:String, required:true } } } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12
| import Vue from 'vue'
import App from './App.vue'
Vue.config.productionTip = false
new Vue({ el:'#app', render: h => h(App) })
|
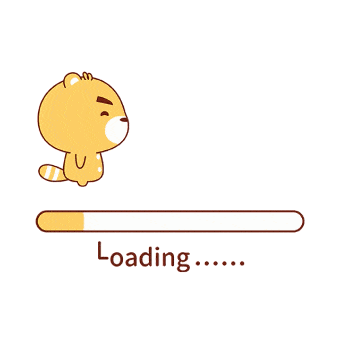
mixin(混入)
mixin:属性和方法以组件自身的为主 生命周期不以任何人为主都要 (混合在前)
- 功能:可以把多个组件共用的配置提取成一个混入对象
- 使用方式:
第一步定义混合:1 2 3 4 5
| { data(){....}, methods:{....} .... }
|
第二步使用混入:
全局混入:Vue.mixin(xxx)
局部混入:mixins:['xxx']
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| <template> <div> <h2 @click="showName">学生姓名:{{name}}</h2> <h2>学生性别:{{sex}}</h2> </div> </template>
<script>
export default { name:'Student', data() { return { name:'张三', sex:'男' } }, } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
| <template> <div> <h2 @click="showName">学校名称:{{name}}</h2> <h2>学校地址:{{address}}</h2> </div> </template>
<script>
export default { name:'School', data() { return { name:'尚硅谷', address:'北京', x:666 } }, } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <template> <div> <School/> <hr> <Student/> </div> </template>
<script> import School from './components/School' import Student from './components/Student'
export default { name:'App', components:{School,Student} } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| import Vue from 'vue'
import App from './App.vue' import {hunhe,hunhe2} from './mixin'
Vue.config.productionTip = false
Vue.mixin(hunhe) Vue.mixin(hunhe2)
new Vue({ el:'#app', render: h => h(App) })
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| export const hunhe = { methods: { showName(){ alert(this.name) } }, mounted() { console.log('你好啊!') }, } export const hunhe2 = { data() { return { x:100, y:200 } }, }
|
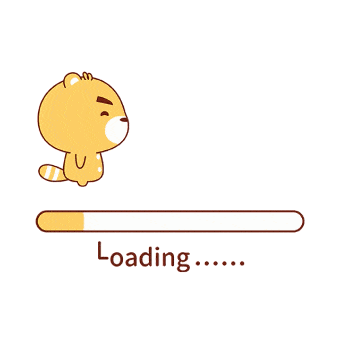
Vue自定义插件
1. 功能:用于增强Vue
2. 本质:包含install
方法的一个对象,install的第一个参数是Vue
,第二个以后的参数是插件使用者传递的数据
。
3. 定义插件:
1 2 3 4 5 6 7 8 9 10 11 12 13 14
| 对象.install = function (Vue, options) { Vue.filter(....)
Vue.directive(....)
Vue.mixin(....)
Vue.prototype.$myMethod = function () {...} Vue.prototype.$myProperty = xxxx }
|
4. 使用插件:Vue.use()
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29
| ```
- <font size="4" face="华文中宋">School.vue
```Javascript <template> <div> <h2>学校名称:{{name | mySlice}}</h2> <h2>学校地址:{{address}}</h2> <button @click="test">点我测试一个hello方法</button> </div> </template>
<script> export default { name:'School', data() { return { name:'尚硅谷atguigu', address:'北京', } }, methods: { test(){ this.hello() } }, } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19
| <template> <div> <h2>学生姓名:{{name}}</h2> <h2>学生性别:{{sex}}</h2> <input type="text" v-fbind:value="name"> </div> </template>
<script> export default { name:'Student', data() { return { name:'张三', sex:'男' } }, } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18
| <template> <div> <School/> <hr> <Student/> </div> </template>
<script> import School from './components/School' import Student from './components/Student'
export default { name:'App', components:{School,Student} } </script>
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| import Vue from 'vue'
import App from './App.vue'
import plugins from './plugins'
Vue.config.productionTip = false
Vue.use(plugins,1,2,3)
new Vue({ el:'#app', render: h => h(App) })
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| export default { install(Vue,x,y,z){ console.log(x,y,z) Vue.filter('mySlice',function(value){ return value.slice(0,4) })
Vue.directive('fbind',{ bind(element,binding){ element.value = binding.value }, inserted(element,binding){ element.focus() }, update(element,binding){ element.value = binding.value } })
Vue.mixin({ data() { return { x:100, y:200 } }, })
Vue.prototype.hello = ()=>{alert('你好啊')} } }
|
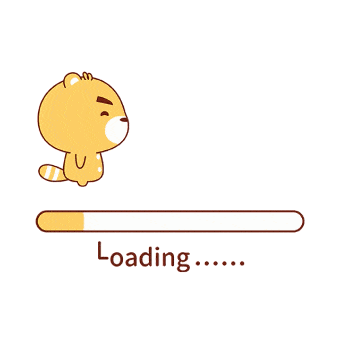